ブログ
これまでに経験してきたプロジェクトで気になる技術の情報を紹介していきます。
Vue3でMapboxを使用する - マーカとポップアップ編 -
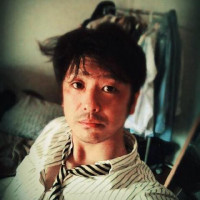
マーカを表示する
基本のマーカ
onMounted
のなかに追加
// 基本のマーカ
const marker = new mapboxgl.Marker()
.setLngLat([135.4262, 34.8215])
.addTo(map);
ドラッガブル
あとで位置情報を取りたいのでリアクティブにしておく
トップレベルに追加
// marker インスタンス
let targetMarker = null;
//...
// ドラッガブルマーカのいち情報
const targetInfo = reactive({ lng: 135.4260, lat: 34.8201 });
onMounted
のなかに追加
// ドラッガブル
targetMarker = new mapboxgl.Marker({
color: "tomato",
draggable: true,
})
.setLngLat([targetInfo.lng, targetInfo.lat])
.addTo(map);
いろんな設定
onMounted
のなかに追加
// いろんな設定
const oliveMarker = new mapboxgl.Marker({
// iconの色
color: "olive", // default: '#3FB1CE'
// 100pxドラッグしてから初めて動く
clickTolerance: 100, // default: 0
// ドラッグ
draggable: true, // default: false
// iconの回転
rotation: 50, // default: 0
// iconの大きさ
scale: 1.5, // default: 1 (41px and a width of 27px)
})
.setLngLat([135.4254, 34.8221])
.addTo(map);
アンカーの位置を変更
onMounted
のなかに追加
// アンカー位置を変更
const orangeMarker1 = new mapboxgl.Marker({
color: "orange",
anchor: "top-left", // default: 'center' [top, bottom, left, right, top-left, top-right, bottom-left', bottom-right]
scale: 0.5,
})
.setLngLat([135.4268, 34.8201])
.addTo(map);
const orangeMarker2 = new mapboxgl.Marker({
color: "orange",
anchor: "center", // default: 'center' [top, bottom, left, right, top-left, top-right, bottom-left', bottom-right]
scale: 0.5,
})
.setLngLat([135.4268, 34.8201])
.addTo(map);
const orangeMarker3 = new mapboxgl.Marker({
color: "orange",
anchor: "bottom-right", // default: 'center' [top, bottom, left, right, top-left, top-right, bottom-left', bottom-right]
scale: 0.5,
})
.setLngLat([135.4268, 34.8201])
.addTo(map);
カスタム
カスタムマーカの要素を作るファンクションを作成
トップレベルに追加
const createMarkerElm = (src, callback = null) => {
const el = document.createElement("img");
el.className = "image-marker";
el.src = src;
let style = "";
style += "border-radius: 50%;";
style += "object-fit: cover;";
style += "border-radius: 50%;";
style += "width: 40px;";
style += "height: 40px;";
el.setAttribute("style", style);
el.addEventListener("click", () => {
callback();
});
return el;
};
onMounted
のなかに追加
// カスタムマーカ
var markerElm = createMarkerElm("https://placekitten.com/g/40/40", () => {
alert("hello!");
});
const catMarker = new mapboxgl.Marker({
element: markerElm,
})
.setLngLat([135.4276, 34.8225])
.addTo(map);
ポップアップ付き
onMounted
のなかに追加
// ポップアップ付き
var popupElm = createPopupElm('hello!')
const chocolateMarker = new mapboxgl.Marker({
color: "chocolate",
})
.setLngLat([135.4248, 34.8200])
.setPopup(new mapboxgl.Popup().setHTML(popupElm)) // add popup
.addTo(map);
ポップアップを表示する
基本のポップアップ
onMounted
のなかに追加
const popup = new mapboxgl.Popup()
.setLngLat([135.4255, 34.8190])
.setHTML(popupElm)
.addTo(map);
クローズボタンなし、クラス付き
const lavenderPopup = new mapboxgl.Popup({closeButton: false, className: 'lavender-popup'})
.setLngLat([135.4263, 34.8190])
.setHTML('lavender!')
.addTo(map);
スタイルをグローバルについか追加
<style>
.lavender-popup .mapboxgl-popup-content {
background-color: lavender;
}
.lavender-popup.mapboxgl-popup-anchor-left .mapboxgl-popup-tip {
border-right-color: lavender;
}
.lavender-popup.mapboxgl-popup-anchor-right .mapboxgl-popup-tip {
border-left-color: lavender;
}
.lavender-popup.mapboxgl-popup-anchor-bottom .mapboxgl-popup-tip {
border-top-color: lavender;
}
.lavender-popup.mapboxgl-popup-anchor-top .mapboxgl-popup-tip {
border-bottom-color: lavender;
}
</style>
カスタムポップアップ
ポップアップの要素を作るファンクションを作成
トップレベルに追加
const createPopupElm = (message) => {
// なんでかできない。。
// const el = document.createElement("div");
// el.className = "popup";
// el.textContent = message;
// let style = "";
// style += "padding: 1ch 1ch 0ch 1ch;";
// el.setAttribute("style", style);
return `<div class="custom-popup" style="padding: 1ch 2ch 0ch;">${message}</div>`;
};
onMounted
のなかに追加
var popupElm = createPopupElm("hello");
const popup = new mapboxgl.Popup({ closeButton: false })
.setLngLat([135.4270, 34.8190])
.setHTML(popupElm)
.addTo(map);
スタイルをグローバルについか追加
.thistle-popup .mapboxgl-popup-content {
background-color: thistle;
}
.thistle-popup.mapboxgl-popup-anchor-left .mapboxgl-popup-tip {
border-right-color: thistle;
}
.thistle-popup.mapboxgl-popup-anchor-right .mapboxgl-popup-tip {
border-left-color: thistle;
}
.thistle-popup.mapboxgl-popup-anchor-bottom .mapboxgl-popup-tip {
border-top-color: thistle;
}
.thistle-popup.mapboxgl-popup-anchor-top .mapboxgl-popup-tip {
border-bottom-color: thistle;
}
.custom-popup {
font-size: large;
}
Vue3でMapboxを使用する - マーカとポップアップ編 -
Vue3でMapboxを使用する - マーカとポップアップ編 -
2022-03-05 18:10:49
2022-03-05 18:25:41
コメントはありません。